Download the PHP package spatie/laravel-package-tools without Composer
On this page you can find all versions of the php package spatie/laravel-package-tools. It is possible to download/install these versions without Composer. Possible dependencies are resolved automatically.
Download spatie/laravel-package-tools
More information about spatie/laravel-package-tools
Files in spatie/laravel-package-tools
Package laravel-package-tools
Short Description Tools for creating Laravel packages
License MIT
Homepage https://github.com/spatie/laravel-package-tools
Informations about the package laravel-package-tools
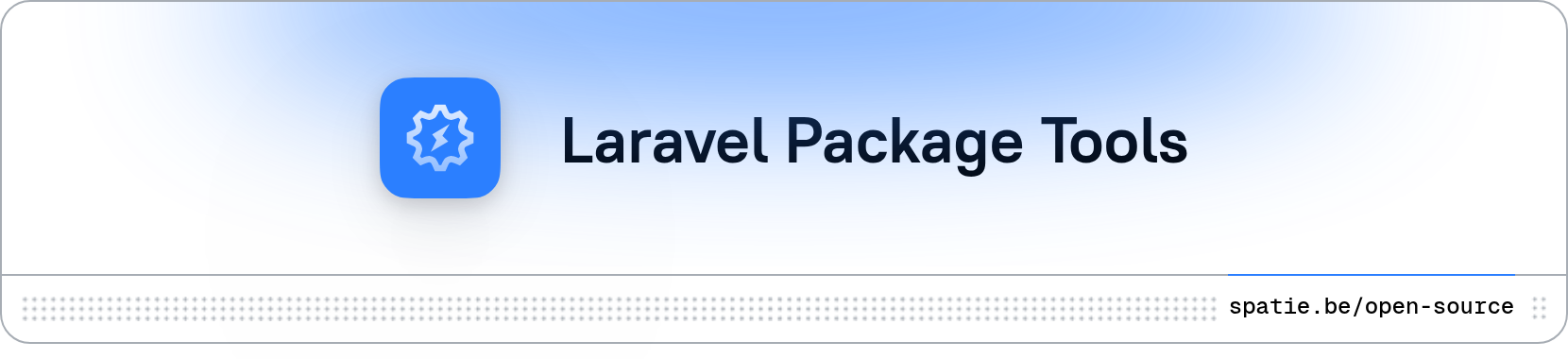
Tools for creating Laravel packages
[](https://packagist.org/packages/spatie/laravel-package-tools)  [](https://packagist.org/packages/spatie/laravel-package-tools)This package contains a PackageServiceProvider
that you can use in your packages to easily register config files,
migrations, and more.
Here's an example of how it can be used.
Under the hood it will do the necessary work to register the necessary things and make all sorts of files publishable.
Support us
We invest a lot of resources into creating best in class open source packages. You can support us by buying one of our paid products.
We highly appreciate you sending us a postcard from your hometown, mentioning which of our package(s) you are using. You'll find our address on our contact page. We publish all received postcards on our virtual postcard wall.
Getting started
This package is opinionated on how you should structure your package. To get started easily, consider
using our package-skeleton repo to start your package. The
skeleton is structured perfectly to work perfectly with the PackageServiceProvider
in this package.
Usage
To avoid needing to scroll through to find the right usage section, here is a Table of Contents:
- Directory Structure
- Making your functionality publishable
- Getting Started
- Assets
- Blade Components
- Blade Anonymous Components
- Blade Custom Directives
- Blade Custom Echo Handlers
- Blade Custom Conditionals
- Commands - Callable and Console
- Optimize Commands (Laravel v11+)
- Config Files
- Events & Listeners
- Inertia Components
- Livewire Views and Components
- Database Migrations
- Routes
- Publishable Service Providers
- Translations
- Views
- View Composers
- Views Global Shared Data
- Creating and Install Command
- Lifecycle Hooks
Directory Structure
This package is opinionated on how you should structure your package, and by default expects a structure based on our package-skeleton repo, and to get started easily you should consider using this to start your package.
The structure for a package expected by default looks like this:
Note: When using paths in any Package method except discoversMigrations()
,
the path given is relative to the location of your primary Service Provider
i.e. relative to <package root>/src
so e.g. <package root>/ConfigFiles
would be specified as ../ConfigFiles
.
Getting Started
In your package you should let your service provider extend Spatie\LaravelPackageTools\PackageServiceProvider
.
Defining your package name with a call to name()
is mandatory.
Note: If your package name starts with laravel-
then this prefix will be omitted
and the remainder of the name used as a short-name instead when publishing files etc.
And now let's look at all the different Laravel functions this supports...
assing the package name to name
is mandatory.
Assets
Any assets your package provides, should be placed in the <package root>/resources/dist/
directory.
You can make these assets publishable the hasAssets
method.
Users of your package will be able to publish the assets with this command:
This will copy over the assets to the public/vendor/<your-package-name>
directory in the app where your package is
installed in.
Blade view components
Any Blade view components that your package provides should be placed in the <package root>/src/Components
directory.
You can register these views with the hasViewComponents
command.
This will register your view components with Laravel. In the case of Alert::class
, it can be referenced in views
as <x-spatie-alert />
, where spatie
is the prefix you provided during registration.
Calling hasViewComponents
will also make view components publishable, and will be published
to app/Views/Components/vendor/<package name>
.
Users of your package will be able to publish the view components with this command:
Commands - Callable and Console
You can register any command you package provides with the hasCommand
function.
`
If your package provides multiple commands, you can either use hasCommand
multiple times, or pass an array
to hasCommands
Config Files
To register a config file, you should create a php file with your package name in the config
directory of your
package. In this example it should be at <package root>/config/your-package-name.php
.
If your package name starts with laravel-
, we expect that your config file does not contain that prefix. So if your
package name is laravel-cool-package
, the config file should be named cool-package.php
.
To register that config file, call hasConfigFile()
on $package
in the configurePackage
method.
The hasConfigFile
method will also make the config file publishable. Users of your package will be able to publish the
config file with this command.
Should your package have multiple config files, you can pass their names as an array to hasConfigFile
Inertia Components
Any .vue
or .jsx
files your package provides, should be placed in the <package root>/resources/js/Pages
directory.
You can register these components with the hasInertiaComponents
command.
This will register your components with Laravel.
The user should publish the inertia components manually or using the installer-command in order to use them.
If you have an inertia component <package root>/resources/js/Pages/myComponent.vue
, you can use it like
this: Inertia::render('YourPackageName/myComponent')
. Of course, you can also use subdirectories to organise your components.
Publishing inertia components
Calling hasInertiaComponents
will also make inertia components publishable. Users of your package will be able to publish the views with this
command:
Also, the inertia components are available in a convenient way with your package installer-command
Working with migrations
The PackageServiceProvider
assumes that any migrations are placed in this
directory: <package root>/database/migrations
. Inside that directory you can put any migrations.
To register your migration, you should pass its name without the extension to the hasMigration
table.
If your migration file is called create_my_package_tables.php.stub
you can register them like this:
Should your package contain multiple migration files, you can just call hasMigration
multiple times or
use hasMigrations
.
Alternatively, if you wish to publish all migrations in your package by default, you may call discoversMigrations
.
Calling this method will look for migrations in the ./database/migrations
directory of your project. However, if you have defined your migrations
in a different folder, you may pass a value to the $path
variable to instruct the app to discover migrations from that location.
Calling either hasMigration
, hasMigration
or discoversMigrations
will also make migrations publishable. Users of your package will be able to publish the
migrations with this command:
Like you might expect, published migration files will be prefixed with the current datetime.
You can also enable the migrations to be registered without needing the users of your package to publish them:
Routes
The PackageServiceProvider
assumes that any route files are placed in this directory: <package root>/routes
. Inside
that directory you can put any route files.
To register your route, you should pass its name without the extension to the hasRoute
method.
If your route file is called web.php
you can register them like this:
Should your package contain multiple route files, you can just call hasRoute
multiple times or use hasRoutes
.
Publishable Service Providers
Some packages need an example service provider to be copied into the app\Providers
directory of the Laravel app. Think
of for instance, the laravel/horizon
package that copies an HorizonServiceProvider
into your app with some sensible
defaults.
The file that will be copied to the app should be stored in your package
in /resources/stubs/{$nameOfYourServiceProvider}.php.stub
.
When your package is installed into an app, running this command...
... will copy /resources/stubs/{$nameOfYourServiceProvider}.php.stub
in your package
to app/Providers/{$nameOfYourServiceProvider}.php
in the app of the user.
Translations
Any translations your package provides, should be placed in the <package root>/resources/lang/<language-code>
directory.
You can register these translations with the hasTranslations
command.
This will register the translations with Laravel.
Assuming you save this translation file at <package root>/resources/lang/en/translations.php
...
... your package and users will be able to retrieve the translation with:
If your package name starts with laravel-
then you should leave that off in the example above.
Coding with translation strings as keys, you should create JSON files
in <package root>/resources/lang/<language-code>.json
.
For example, creating <package root>/resources/lang/it.json
file like so:
...the output of...
...will be Ciao!
if the application uses the Italian language.
Calling hasTranslations
will also make translations publishable. Users of your package will be able to publish the
translations with this command:
Views
Any views your package provides, should be placed in the <package root>/resources/views
directory.
You can register these views with the hasViews
command.
This will register your views with Laravel.
If you have a view <package root>/resources/views/myView.blade.php
, you can use it like
this: view('your-package-name::myView')
. Of course, you can also use subdirectories to organise your views. A view
located at <package root>/resources/views/subdirectory/myOtherView.blade.php
can be used
with view('your-package-name::subdirectory.myOtherView')
.
Using a custom view namespace
You can pass a custom view namespace to the hasViews
method.
You can now use the views of the package like this:
Publishing the views
Calling hasViews
will also make views publishable. Users of your package will be able to publish the views with this
command:
Note:
If you use custom view namespace then you should change your publish command like this:
View Composers
You can register any view composers that your project uses with the hasViewComposers
method. You may also register a
callback that receives a $view
argument instead of a classname.
To register a view composer with all views, use an asterisk as the view name '*'
.
Views Global Shared Data
You can share data with all views using the sharesDataWithAllViews
method. This will make the shared variable
available to all views.
Creating an Install Command
Instead of letting your users manually publishing config files, migrations, and other files manually, you could opt to add an install command that does all this work in one go. Packages like Laravel Horizon and Livewire provide such commands.
When using Laravel Package Tools, you don't have to write an InstallCommand
yourself. Instead, you can simply
call, hasInstallCommand
and configure it using a closure. Here's an example.
With this in place, the package user can call this command:
Using the code above, that command will:
- publish the config file
- publish the assets
- publish the migrations
- copy the
/resources/stubs/MyProviderName.php.stub
from your package toapp/Providers/MyServiceProviderName.php
, and also register that provider inconfig/app.php
- ask if migrations should be run now
- prompt the user to open up
https://github.com/'your-vendor/your-repo-name'
in the browser in order to star it
You can also call startWith
and endWith
on the InstallCommand
. They will respectively be executed at the start and
end when running php artisan your-package-name:install
. You can use this to perform extra work or display extra
output.
Lifecycle Hooks
You can put any custom logic your package needs while starting up in one of these methods:
registeringPackage
: will be called at the start of theregister
method ofPackageServiceProvider
packageRegistered
: will be called at the end of theregister
method ofPackageServiceProvider
bootingPackage
: will be called at the start of theboot
method ofPackageServiceProvider
packageBooted
: will be called at the end of theboot
method ofPackageServiceProvider
Testing
This package now supports test groups as follows:
The current groups suported are:
- base
- assets
- blade
- commands
- config
- inertia
- migrations
- provider
- routes
- shareddata
- translations
- viewcomposer
- views
- installer
Additionally, if you wish to test only backwards compatibility you can use:
- legacy
Note: InvalidPackage
exceptions thrown during Laravel application bootup are reported by Pest,
but because the occur before the start of a test case
Pest by default does not allow you intentionally to test for them being thrown.
The tests in this package now include checks for intentional InvalidPackage
exceptions being thrown
by catching and saving such exceptions in the TestServiceProvider,
and then rethrowing the exception at the very start of a Pest test case,
and this is achieved by loading a modified version of the Pest test()
function
before anything else is loaded.
Whilst this is done for you if you run composer test
,
if you want to run vendor/bin/pest
directly you now need to run it like this:
Changelog
Please see CHANGELOG for more information on what has changed recently.
Contributing
Please see CONTRIBUTING for details.
Security Vulnerabilities
Please review our security policy on how to report security vulnerabilities.
Credits
- Freek Van der Herten
- All Contributors
License
The MIT License (MIT). Please see License File for more information.