Download the PHP package ackintosh/ganesha without Composer
On this page you can find all versions of the php package ackintosh/ganesha. It is possible to download/install these versions without Composer. Possible dependencies are resolved automatically.
Download ackintosh/ganesha
More information about ackintosh/ganesha
Files in ackintosh/ganesha
Package ganesha
Short Description PHP implementation of Circuit Breaker pattern
License MIT
Informations about the package ganesha
Ganesha
Ganesha is PHP implementation of Circuit Breaker pattern which has multi strategies to avoid cascading failures and supports various storages to record statistics.
This is one of the Circuit Breaker implementations in PHP that is actively developed, production-ready, well-tested and well-documented. :muscle: You can easily integrate Ganesha into your existing code base, as Ganesha provides just simple interfaces and Guzzle Middleware behaves transparency.
Table of contents
- Ganesha
- Table of contents
- Are you interested?
- Unveil Ganesha
- Usage
- Strategies
- Adapters
- Customizing storage keys
- Ganesha :heart: Guzzle
- Ganesha :heart: OpenAPI Generator
- Ganesha :heart: Symfony HttpClient
- Companies using Ganesha :rocket:
- The articles/videos Ganesha loves :sparkles: :elephant: :sparkles:
- Run tests
- Requirements
- Build promotion site with Soushi
- Author
Are you interested?
Here is an example which shows you how Ganesha behaves when a failure occurs.
It is easily executable. All you need is Docker.
Unveil Ganesha
Usage
Ganesha provides following simple interfaces. Each method receives a string (named $service
in example) to identify the service. $service
will be the service name of the API, the endpoint name, etc. Please remember that Ganesha detects system failure for each $service
.
Three states of circuit breaker
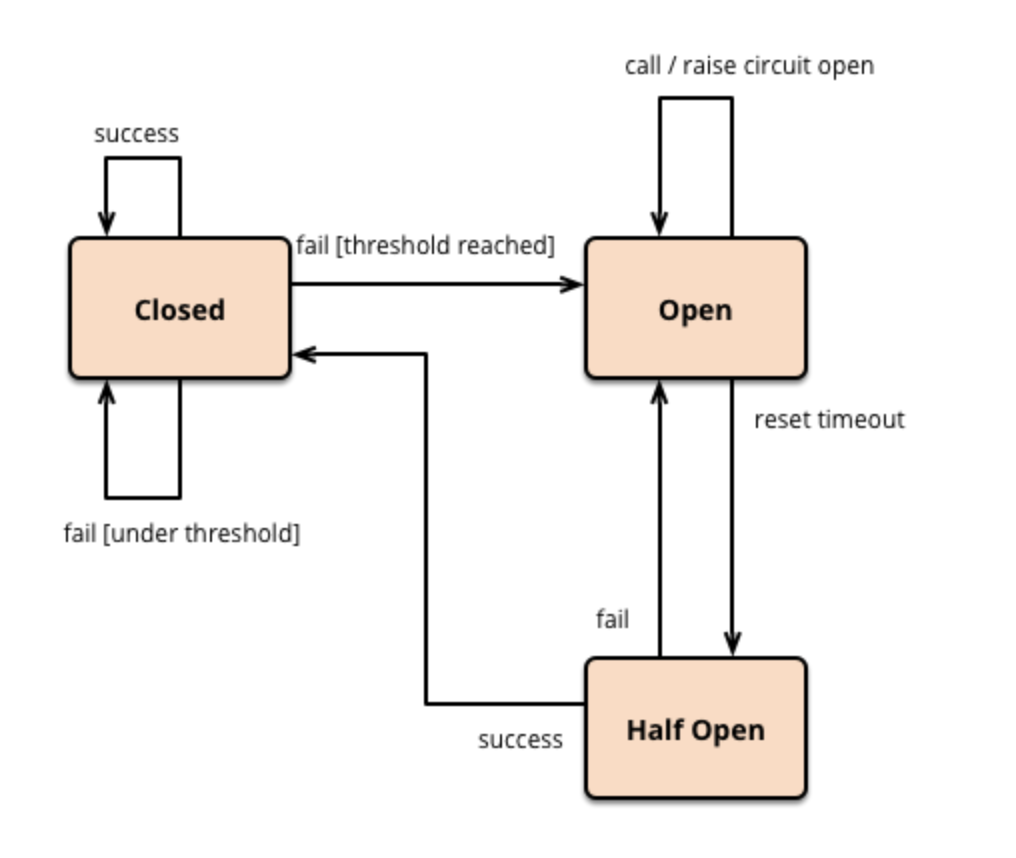
(martinfowler.com : CircuitBreaker)
Ganesha follows the states and transitions described in the article faithfully. $ganesha->isAvailable()
returns true
if the circuit states on Closed
, otherwise it returns false
.
Subscribe to events in ganesha
- When the circuit state transitions to
Open
the eventGanesha::EVENT_TRIPPED
is triggered - When the state back to
Closed
the eventGanesha::EVENT_CALMED_DOWN
is triggered
Disable
If disabled, Ganesha keeps to record success/failure statistics, but Ganesha doesn't trip even if the failure count reached to a threshold.
Reset
Resets the statistics saved in a storage.
Strategies
Ganesha has two strategies which avoids cascading failures.
Rate
Note about "time window": The Storage Adapter implements either SlidingTimeWindow or TumblingTimeWindow. The difference of the implementation comes from constraints of the storage functionalities.
[SlidingTimeWindow]
- SlidingTimeWindow implements a time period that stretches back in time from the present. For instance, a SlidingTimeWindow of 30 seconds includes any events that have occurred in the past 30 seconds.
- Redis adapter and MongoDB adapter implements SlidingTimeWindow.
The details to help us understand visually is shown below:
(quoted from Introduction to Stream Analytics windowing functions - Microsoft Azure)
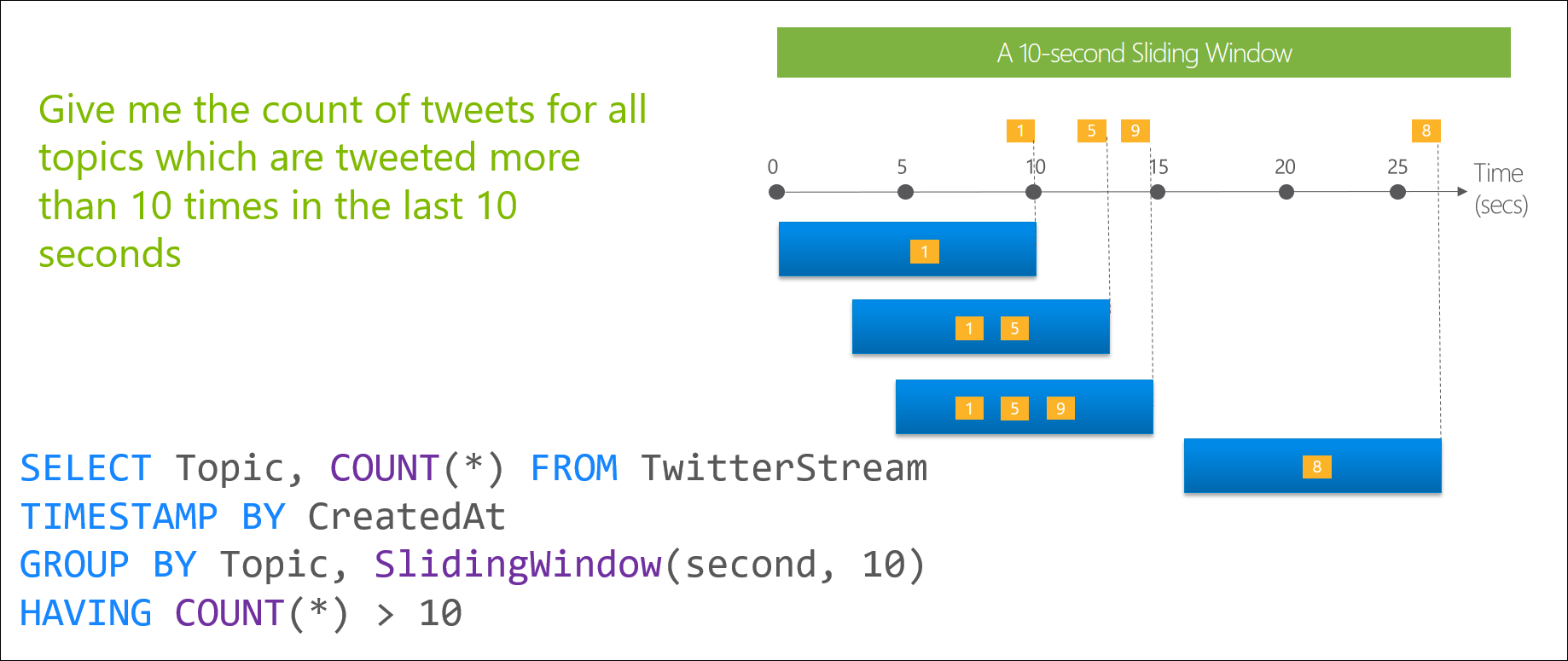
[TumblingTimeWindow]
- TumblingTimeWindow implements time segments, which are divided by a value of
timeWindow
. - APCu adapter and Memcached adapter implement TumblingTimeWindow.
The details to help us understand visually is shown below:
(quoted from Introduction to Stream Analytics windowing functions - Microsoft Azure)
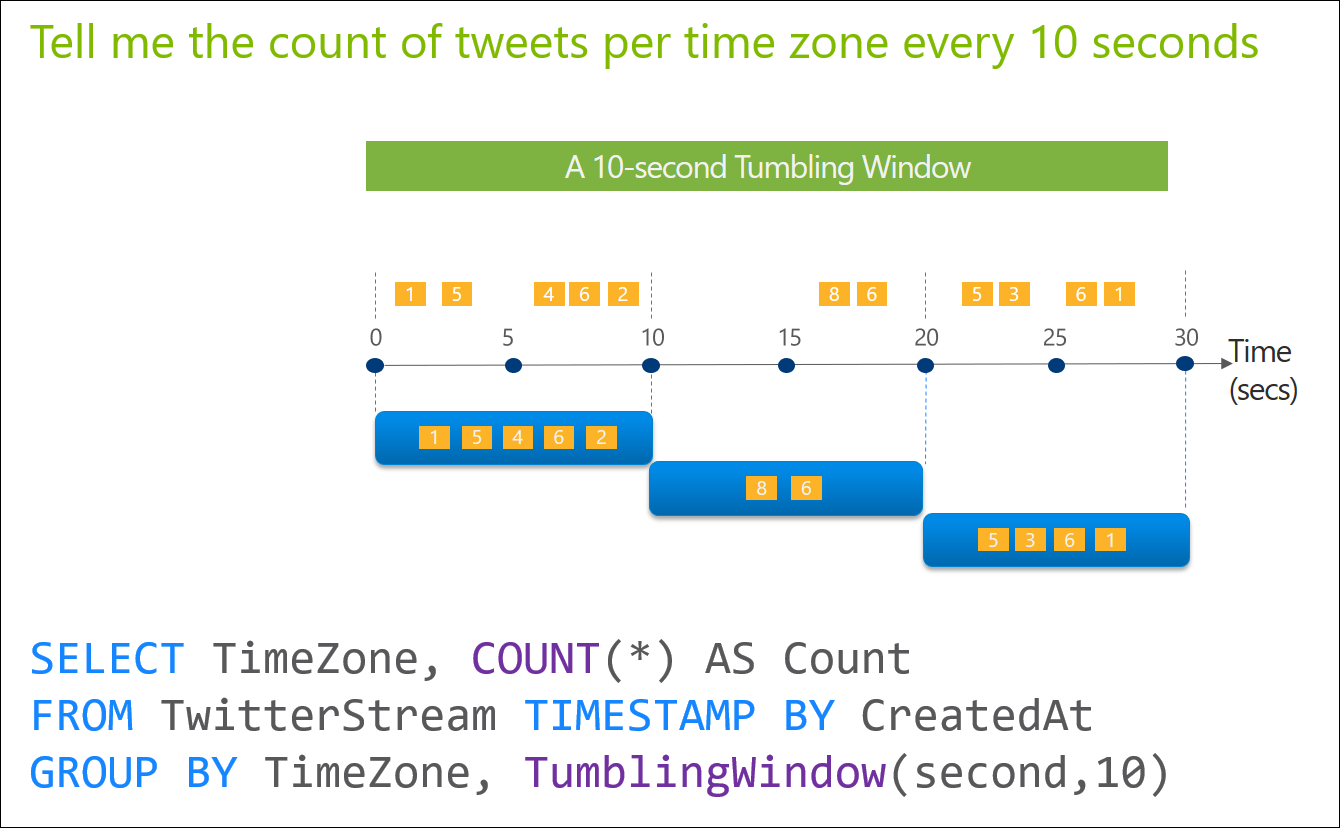
Count
If you prefer the Count strategy use Builder::withCountStrategy()
to build an instance.
Adapters
APCu
The APCu adapter requires the APCu extension.
Note: APCu is internal to each server/instance, not pooled like most Memcache and Redis setups. Each worker's circuit breaker will activate or reset individually, and failure thresholds should be set lower to compensate.
Redis
Redis adapter requires phpredis or Predis client instance. The example below is using phpredis.
Memcached
Memcached adapter requires memcached (NOT memcache) extension.
MongoDB
MongoDB adapter requires mongodb extension.
Customizing storage keys
If you want to customize the keys to be used when storing circuit breaker information, set an instance which implements StorageKeysInterface.
Ganesha :heart: Guzzle
If you are using Guzzle (v6 or higher), Guzzle Middleware powered by Ganesha makes it easy to integrate Circuit Breaker to your existing code base.
How does Guzzle Middleware determine the $service
?
As documented in Usage, Ganesha detects failures for each $service
. Below, We will show you how Guzzle Middleware determine $service
and how we specify $service
explicitly.
By default, the host name is used as $service
.
You can also specify $service
via a option passed to client, or request header. If both are specified, the option value takes precedence.
Alternatively, you can apply your own rules by implementing a class that implements the ServiceNameExtractorInterface
.
How does Guzzle Middleware determine the failure?
By default, if the next handler promise is fulfilled ganesha will consider it a success, and a failure if it is rejected.
You can implement your own rules on fulfilled response by passing an implementation of FailureDetectorInterface
to the middleware.
Ganesha :heart: OpenAPI Generator
PHP client generated by OpenAPI Generator is using Guzzle as HTTP client and as we mentioned as Ganesha :heart: Guzzle, Guzzle Middleware powered by Ganesha is ready. So it is easily possible to integrate Ganesha and the PHP client generated by OpenAPI Generator in a smart way as below.
Ganesha :heart: Symfony HttpClient
If you are using Symfony HttpClient, GaneshaHttpClient makes it easy to integrate Circuit Breaker to your existing code base.
How does GaneshaHttpClient determine the $service
?
As documented in Usage, Ganesha detects failures for each $service
. Below, We will show you how GaneshaHttpClient determine $service
and how we specify $service
explicitly.
By default, the host name is used as $service
.
You can also specify $service
via a option passed to client, or request header. If both are specified, the option value takes precedence.
Alternatively, you can apply your own rules by implementing a class that implements the ServiceNameExtractorInterface
.
How does GaneshaHttpClient determine the failure?
As documented in Usage, Ganesha detects failures for each $service
.
Below, We will show you how GaneshaHttpClient specify failure explicitly.
By default, Ganesha considers a request is successful as soon as the server responded, whatever the HTTP status code.
Alternatively, you can use the RestFailureDetector
implementation of FailureDetectorInterface
to specify a list of HTTP Status Code to be considered as failure via an option passed to client.
This implementation will consider failure when these HTTP status codes are returned by the server:
- 500 (Internal Server Error)
- 502 (Bad Gateway or Proxy Error)
- 503 (Service Unavailable)
- 504 (Gateway Time-out)
- 505 (HTTP Version not supported)
Alternatively, you can apply your own rules by implementing a class that implements the FailureDetectorInterface
.
Companies using Ganesha :rocket:
Here are some companies using Ganesha in production! We are proud of them. :elephant:
To add your company to the list, please visit README.md and click on the icon to edit the page or let me know via issues/twitter.
(alphabetical order)
The articles/videos Ganesha loves :sparkles: :elephant: :sparkles:
Here are some articles/videos introduce Ganesha! All of them are really shining like a jewel for us. :sparkles:
Articles
- 2025-02-05 Unorthodox Monoliths in Laravel | Studocu Tech
- 2024-02-13 Webhooks at scale @Yousign. Yousign s’appuie sur des webhooks pour… | by Fabien Paitry | Yousign Engineering & Product
- 2022-09-02 Using a circuit breaker to spare the API we are calling | Bedrock Tech Blog
- 2021-06-25 Чек-лист: как оставаться отказоустойчивым, переходя на микросервисы на PHP (и как правильно падать) / Блог компании Skyeng / Хабр
- 2020-12-21 장애 확산을 막기 위한 서킷브레이커 패턴을 PHP에서 구현해보자
- 2020-04-22 PHP Annotated – April 2020 | PhpStorm Blog
- 2020-03-23 Circuit Breaker - SarvenDev
- 2020-03-23 PHP-Дайджест № 177 (23 марта – 6 апреля 2020) / Хабр
- 2019-08-01 PHP Weekly. Archive. August 1, 2019. News, Articles and more all about PHP
- 2019-07-15 PHP Annotated – July 2019 | PhpStorm Blog
- 2019-04-25 PHP Weekly. Archive. April 25, 2019. News, Articles and more all about PHP
- 2019-03-18 A Semana PHP - Edição Nº229 | Revue
- 2018-06-08 Безопасное взаимодействие в распределенных системах / Блог компании Badoo / Хабр
- 2018-01-22 PHP DIGEST #12: NEWS & TOOLS (JANUARY 1 - JANUARY 14, 2018)
Videos
Run tests
We can run unit tests on a Docker container, so it is not necessary to install the dependencies in your machine.
Requirements
- An extension or client library which is used by the storage adapter you've choice will be required. Please check the Adapters section for details.
Version Guidance
Version | PHP Version | Latest Release |
---|---|---|
4.x | >=8.1 | Latest Release |
3.x | >=8.0 | 3.2.0 |
2.x | >=7.3 | 2.0.2 |
1.x | >=7.1 | 1.3.0 |
0.x | >=5.6 | 0.5.0 |
Author
Ganesha © ackintosh, Released under the MIT License.
Authored and maintained by ackintosh
GitHub @ackintosh / Twitter @NAKANO_Akihito / Blog (ja)